As a programmer, we should know the importance of breaking down complex problems into smaller, more manageable pieces. This is called top down approach, which can simplify your code and make it easier to understand, test, and maintain.
In this article, we’ll show you how to use this approach. Let’s solve a complex geophysics problem: calculating the travel time of a seismic wave through a layered earth model.
Calculating Seismic Travel Time through a Layered Earth Model
Seismic waves are an important tool for geophysicists to study the structure of the Earth’s interior. The travel time of a seismic wave through the Earth’s subsurface can provide valuable information about the properties of the subsurface, such as density and velocity.
The Problem
The velocity of a seismic wave is different in different layers of the earth. To calculate the travel time of a seismic wave, we need to take into account the depth of each layer in the earth model and the velocity of the seismic wave in each layer.
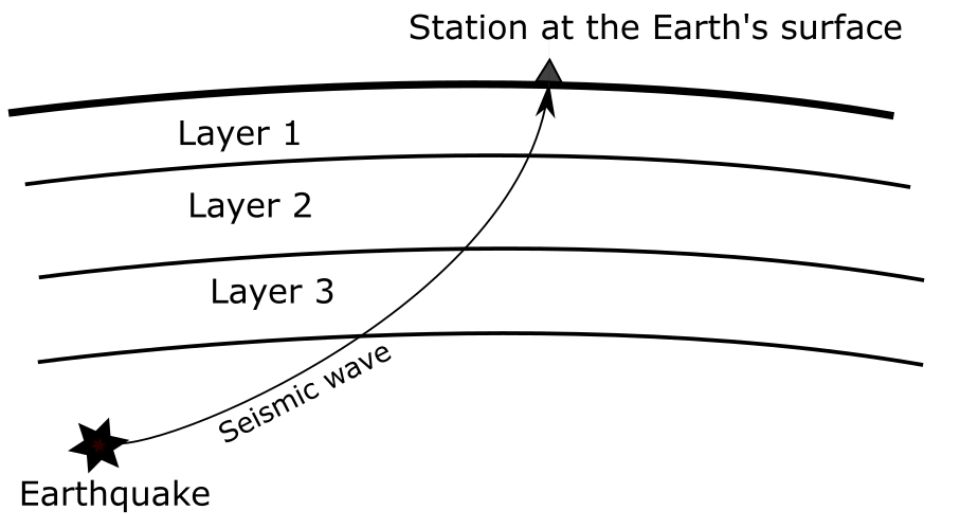
The Solution
To solve this problem, we can use a top-down approach, which starts with the overall problem and breaks it down into smaller and simpler subproblems.
Let’s assume we have the list of the depth of the top boundary of $n$ layers as $0,d_1,d_2…d_n$ starting from the surface, and seismic velocities in these layers are $v_0,v_1,v_3…v_n$. In this case, the subproblems are:
- Calculating the thickness of $i^{th}$ layer in the earth model- $d_{i} – d_{i-1}$
- Determining the velocity of the seismic wave in $i^{th}$ layer- $v_{i-1}$
- Calculating the travel time for each layer by dividing the thickness of the layer by the velocity of the wave in $i^{th}$ layer- $\frac{d_{i} – d_{i-1}}{v_{i-1}}$
- Summing up the travel times of each layer to get the total travel time.
Let’s implement each of these subproblems as a single function in Python:
def travel_time(depth, velocity_model):
time = 0
for i in range(1, len(depth)):
layer_thickness = depth[i] - depth[i-1]
layer_velocity = velocity_model[i-1]
time += layer_thickness / layer_velocity
return time
The travel_time
function takes the depth
array and the velocity_model
array as inputs and returns the calculated travel time. The depth
array represents the depth of the layers in the earth model, and the velocity_model
array represents the velocity of the seismic wave in each layer. The function uses a loop to iterate over the depth
array, and calculates the travel time for each layer by dividing the depth of the layer by the velocity of the wave in that layer. The total travel time is the sum of the travel times of each layer.
Here is an example of how to use the travel_time
function:
depth = [0, 5, 10, 20, 30, 40]
velocity_model = [5, 4, 3, 2, 1]
print(travel_time(depth, velocity_model))
This code will produce the following output of 20.58s
How to use this approach effectively
In order to effectively use the top-down approach, it is important to have a clear understanding of the problem that you are trying to solve. This includes identifying the specific subproblems that need to be solved and the input and output of each subproblem.
Additionally, it is important to plan out the overall structure of the code before beginning to write any actual code. This will help to ensure that the final product is well-organized and easy to understand.
Key benefits of Top down approach:
The top down approach is a powerful method for organizing and structuring scientific code. A few advantages that can be inferred from using the above code are:
- Easier debugging and testing: It allows for easier debugging and testing of the code. By breaking the problem into smaller pieces, it becomes easier to identify the source of any errors or bugs that may be present.
- Reusable and modular code: It allows for the development of code that is more modular and reusable. This makes it easier to update or modify specific sections of the code without affecting the rest of the program.
Conclusion
In this article, we have looked at a very simple example of how to calculate the travel time of a seismic wave through a layered earth model in Python using a top down approach.
Key learnings from the post are:
- Practical implementation: How to calculate the travel time of a seismic wave through a layered earth model in Python using a top down approach.
- Key benefits of using top down approach to scientific programming: Easier debugging and testing, Reusable and modular code.
This article was crafted by a group of experts at eigenplus to ensure it adheres to our strict quality standards. The individuals who contributed to this article are:
Author
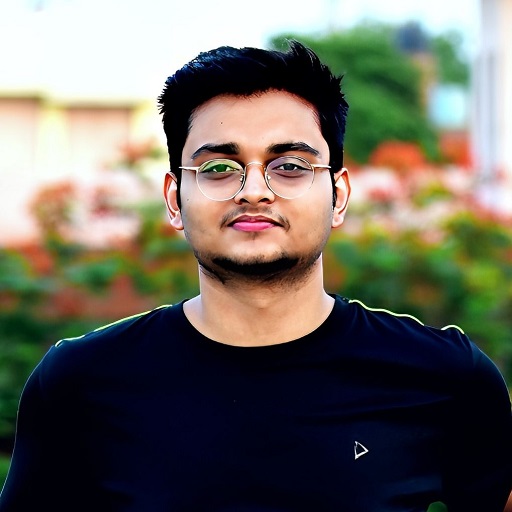
Himanshu Agrawal
MS
He is a PhD scholar in seismology with BS-MS in Geosciences.